Architecture of Nebloc: Part 2 - Code
The second part in a series that aims to describe how nebloc.com is built. This post will outline the frameworks, languages, libraries, and other choices made.
Overview#
The site is made up of two docker images (frontend and backend) and a variety of Google Cloud Platform services.
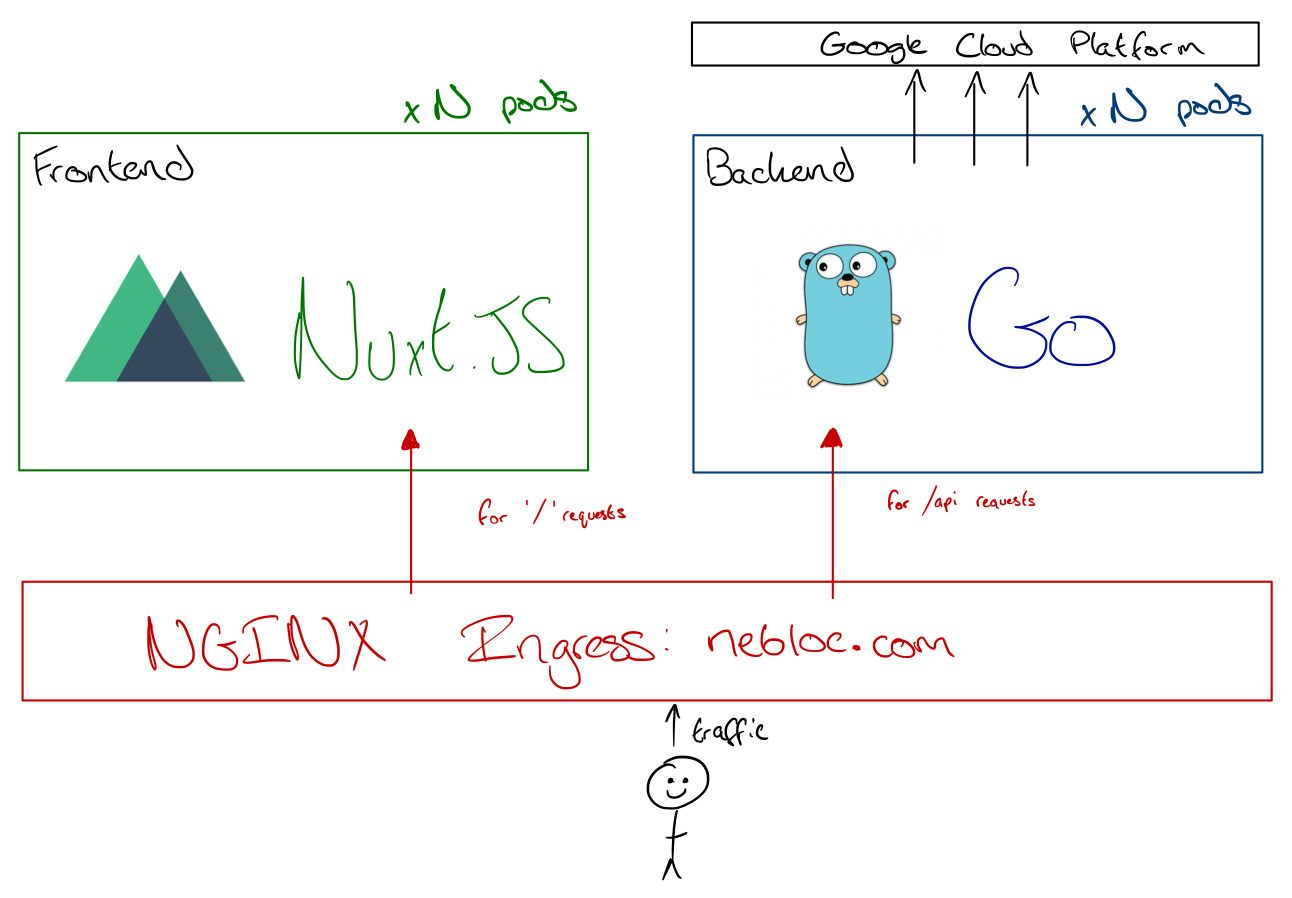
Backend#
The ‘backend’ image is used as a RESTful API server, and mainly serves as a proxy between GCP and the frontend. It returns JSON payloads that contain things such as the list of image URLs for a collection, the description, a list of collections etc.
It is written in The Go Programming language (my current favourite), using the Gin framework as it provides a lot of useful helpers for binding request data, returning JSON, handling errors, etc.
For example, here is part of the handler for retrieving the list of regulars for the about page:
func GetFriends(c *gin.Context) {
// Middleware adds the firestore client to the request
f := c.Value("firestore_client").(*firestore.Client)
friends := &Friends{}
// Omitting code for firestore handling
// Populate `friends` with data
c.JSON(http.StatusOK, friends) // Return JSON representaion of friends
}
Due to being used to the Gin framework and not needing that much work to be done by the backend, it is very simple to maintain and add features to.
Frontend#
The frontend is written in Vue.JS but using Nuxt.JS to speed up development and add Server Side Rendering (SSR) for better SEO performance.
Originally it was pure Vue.JS with Bootstrap 4 CSS library, but after my holiday to Amsterdam was cancelled amidst COVID-19, I spent it implementing Nuxt.JS and decided to change to Bulma as I didn’t like the way it looked in Bootstrap. With Bulma and Buefy implemented the look and feel is a lot cleaner, opting for a white theme to make the photos pop a bit more.
This went in hand with changes to the way images were displayed to all be cropped square, implementation of Flexbox with Bulma columns, and the use @RobinCK’s Vue Gallery package for a more modern user experience.
I have tried to implement the frontend following modern practices; i.e. breaking
bits off into components to make up a page, use of the Vuex store for collection
storage, and use of an api.service
pattern, etc. A lot of inspiration for this
came from looking at the
Vue Real World example.
I have found Vue.JS and Nuxt.JS to be a joy to write (which is surpising because I like to stay away from JS), and although CSS is always annoying, Bulma creates some great looking websites with not a whole lot of work.